Platform API
Squizz.com contains a data Application Programming Interface (API) that allows 3rd party software to send and receive data from the platform. The Data API allows separate organisation business systems to communicate together through the endpoints that the platform's API provides. The API can be used to automate a number of processes, as well as provide ways for organisations to buy and sell within the platform by providing the data it requires. To use the API you will need to have knowledge in building software applications, and in particular on how to make requests over the internet to RESTful web services, which the platform's API is based on.
Prerequisites
Before reading on please ensure that you have understood the following topics:
API Native Programming Language Libraries
The SQUIZZ.com platform's API allows software to be written that directly sends data through its HTTPS endpoint requests, and receive responses in the JSON data format. This however requires additional code to written to send requests to the platform and parse JSON responses. To reduce the code required to be written, open source API libraries for select selected programming languages has been developed. If a native library exists for your programming language listed below we recommend to use that library instead to make calls direct to the API, since it will save software developers time, and effort to develop the ability to push data into and out of the platform. If your programming language is not on the list below visit the SQUIZZ.com feed to talk about your need in developing a library, or otherwise make direct HTTPS calls to the API in the endpoints as listed in the sections below.
SQUIZZ.com Platform API Java Library
This library allows Java applications to call the API using native Java classes and objects. Visit https://github.com/squizzdotcom/squizz-platform-api-java-library to see examples on how to use the library within your Java application, as well as make contributions to the open source library.
SQUIZZ.com Platform API PHP Library
This library allows PHP applications to call the API using native PHP classes and objects.
Visit https://github.com/squizzdotcom/squizz-platform-api-php-library to see examples on how to use the library within your PHP application, as well as make contributions to the open source library.
SQUIZZ.com Platform API .NET Library
This library allows .NET applications to call the API using native .NET classes and objects.
Visit https://github.com/squizzdotcom/squizz-platform-api-dotnet-library to see examples on how to use the library within your .NET application, as well as make contributions to the open source library.
Web Service Endpoint: Create Organisation API Session
The endpoint creates a new session in the API by logging with the credentials of an existing organisation registered in the platform. This endpoint must be called first in order to obtain the session ID that is required for all other organisation endpoints. This endpoint can be also used to only check if an organisation's API credentials given are correct. Ensure that the credentials of the organisation have been setup in the organisation's profile first, which can be set from within the Admin section, within the API Settings dialog.
HTTP Request
HTTP Method | POST | |
HTTP URL | https://api.squizz.com/rest/1/org/create_session | |
Headers | ||
---|---|---|
Content-Type | application/x-www-form-urlencoded | |
Body Parameters | Data Type | Mandatory |
org_id | STRING | Yes |
ID of the organisation issued by squizz.com | ||
api_org_key | STRING | Yes |
Key issued to the organisation by squizz.com to access the API | ||
api_org_pw | STRING | Yes |
Password set by an organisation's administrator person to allow access to the API | ||
create_session | ENUM (Y or N) | No |
If set to N then the credentials will only be verified and the session will not be created. |
HTTP Request Raw Example:
POST https://api.squizz.com/rest/1/org/create_session HTTP/1.1
Host: api.squizz.com
Content-Length: 115
Content-Type: application/x-www-form-urlencoded
org_id=039583957203259WER2484832&api_org_key=12345678901234567890&api_org_pw=exmample_api_password&create_session=Y
HTTP Response
Response Data Type | JSON |
Parameters | Data Type |
---|---|
result | ENUM (SUCCESS or FAILURE) |
Either "SUCCESS" or "FAILURE". If successful then a API session was successfully created. | |
result_code | STRING |
Status code of trying to import the ESD document data. The following codes could be returned:
|
|
session_id | STRING |
ID of the session if one was successfully created. | |
api_version | STRING |
Version of the API being used to handle the endpoint request. |
HTTP Response Example:
Web Service Endpoint: Destroy Organisation API Session
The endpoint terminates an existing session in the API by destroying the it based on the session ID given. Once the API has finished being used it is recommended to destroy the session and close off any resources that it was using on the platform. If the session is not destroyed then eventually it will expire and be forcibly terminated by the platform.
HTTP Request
HTTP Method | GET | |
HTTP URL | https://api.squizz.com/rest/1/org/destroy_session/session_id | |
Parameters | Data Type | Mandatory |
---|---|---|
session_id | STRING | Yes |
ID of the API session. Place the session ID within the URL |
HTTP Response
Response Data Type | JSON |
Parameters | Data Type |
---|---|
result | ENUM (SUCCESS or FAILURE) |
Either "SUCCESS" or "FAILURE". If successful then a API session was successfully destroyed. | |
result_code | STRING |
Status code of trying to destroy the session. See the Appendix I for the list of possible result codes. | |
api_version | STRING |
Version of the API being used to handle the endpoint request. |
HTTP Response Example:
Web Service Endpoint: Validate Organisation API Session
The endpoint checks to see if an existing session still exists when using the API based on the session ID given. This endpoint can be used to verify an existing session exists before requiring to create a new session, or destroy an existing session.
HTTP Request
HTTP Method | GET | |
HTTP URL | https://api.squizz.com/rest/1/org/validate_session/session_id | |
Parameters | Data Type | Mandatory |
---|---|---|
session_id | STRING | Yes |
ID of the API session. Place the session ID within the URL |
HTTP Response
Response Data Type | JSON |
Parameters | Data Type |
---|---|
result | ENUM (SUCCESS or FAILURE) |
Either "SUCCESS" or "FAILURE". If successful then a API session was successfully validated. | |
result_code | STRING |
Status code of trying to validate the session. See the Appendix I for the list of possible result codes. | |
api_version | STRING |
Version of the API being used to handle the endpoint request. | |
session_valid | ENUM (Y or N) |
If set to Y then the session is still valid, otherwise N indicates that it has been destroyed. |
HTTP Response Example:
Web Service Endpoint: Import Organisation Data
The endpoint imports different lists of organisation data based on the given data type. The data being imported must be placed into the Body of the HTTP request in the form of a conforming Ecommerce Standards Document JSON string that matches with the data type specified. For example this endpoint can be used to import a list of product records that have been wrapped up within a JSON formatted "Products Ecommerce Standards Document".Before using this endpoint we recommend that you understand what the Ecommerce Standards Documents are and how you can convert business data from your system into the standards. It is highly recommended that any data uploaded using this endpoint be compressed using the GZIP compression algorithm and uploaded in a stream. Note that there are trading token costs to import the organisation data using this API, of which the organisation importing the data must have enough tokens to allow the data to import. See the Trading Tokens and Pricing Help page for data import costs.
Data Import Types
Below is a list of the following types of documents and records that can be imported into the platform, with the data structured in JSON data conforming to the Ecommerce Standards Documents:
- Taxcodes - Record docs
- Price Levels - Record docs
- Products - Record docs
- Product Price-Level Unit Pricing - Record docs
- Product Price-Level Quantity Based Pricing - Record docs
- Product Customer Account Pricing - Record docs
- Alternate Codes - Attribute Profile Record, Attribute Record, Product Attribute Value Record docs
- Product Stock Quantities - Record docs
- Product Combinations - Combination Profile Record, Combination Profile Field Record, Product Combination Parent Record, Product Combination Record docs
- Attributes - Record docs
- Sales Representatives - Record docs
- Customer Accounts - Record docs
- Supplier Accounts - Record docs
- Customer Account Contracts - Record docs
- Customer Account Addresses - Record docs
- Locations - Record docs
- Purchasers - Record docs
- Surcharges - Record docs
- Payment Types - Record docs
- Sell Units - Record docs
HTTP Request
HTTP Method | POST | |
HTTP URL | https://api.squizz.com/rest/1/org/import_esd/session_id |
|
Parameters | Data Type | Mandatory |
---|---|---|
session_id | STRING | Yes |
ID of the API session. Place the session ID within the URL | ||
import_type_id | INTEGER | Yes |
ID of the data type being imported. Set one of the numbers:
|
||
Body | JSON | Yes |
Body contains a type of Ecommerce Standards Document with one or more records that has been serialized into a JSON data string. |
HTTP Response
Response Data Type | JSON |
Parameters | Data Type |
---|---|
resultStatus | INTEGER |
|
|
configs.result_code | STRING |
Status code of trying to import the organisation's data. The following codes could be returned:
|
|
api_version | STRING |
Version of the API being used to handle the endpoint request. |
HTTP Response Example:
Links
- Platform API Endpoint: Import Organisation Data : Import Price Levels
- Platform API Endpoint: Import Organisation Data : Import Surcharges
- Platform API Endpoint: Import Organisation Data : Import Purchasers
- Platform API Endpoint: Import Organisation Data : Import Makers
- Platform API Endpoint: Import Organisation Data : Import Supplier Accounts
- Platform API Endpoint: Import Organisation Data : Import Related Products - Item Relations
- Platform API Endpoint: Import Organisation Data : Import Maker Model Product Mappings
- Platform API Endpoint: Import Organisation Data : Import Price Levels
- Platform API Endpoint: Import Organisation Data : Import Taxcodes
- Platform API Endpoint: Import Organisation Data : Import Product Customer Account Pricing
- Platform API Endpoint: Import Organisation Data : Import Products
- Platform API Endpoint: Import Organisation Data : Import Attributes, Attribute Profiles and Product Attribute Values
- Platform API Endpoint: Import Organisation Data : Import Sales Representatives
- Platform API Endpoint: Import Organisation Data : Import Product Price-Level Unit Pricing
- Platform API Endpoint: Import Organisation Data : Import Payment Types
- Platform API Endpoint: Import Organisation Data : Import Customer Account Contracts
- Platform API Endpoint: Import Organisation Data : Import Categories
- Platform API Endpoint: Import Organisation Data : Import Product Alternate Codes
- Platform API Endpoint: Import Organisation Data : Import Customer Accounts
- Platform API Endpoint: Import Organisation Data
- Platform API Endpoint: Import Organisation Data : Import Maker Models
- Platform API Endpoint: Import Organisation Data : Import Sales Representatives
- Platform API Endpoint: Import Organisation Data : Import Locations
- Platform API Endpoint: Import Organisation Data : Import Product Price-Level Quantity Based Pricing
- Platform API Endpoint: Import Organisation Data : Import Product Combinations
- Platform API Endpoint: Import Organisation Data : Import Product Stock Quantities
- Platform API Endpoint: Import Organisation Data : Import Supplier Accounts
- Platform API Endpoint: Import Organisation Data : Import Sell Units
Web Service Endpoint: Import Organisation Data Images and Files
Web Service Endpoint: Retrieve Organisation Data
The endpoint allows different kinds of organisational data to be retrieved from another chosen organisation who had previously imported their data into the platform. The endpoint will return a list of records in a conforming Ecommerce Standards document that will be formatted in a JSON HTTP response. For example the endpoint could be called to get a list of products from a supplying organisation, or get a list of stock quantities for the products that a supplier organisation sells. See the list below of the kinds of data that can be retrieved.
Organisations who are supplying the data can control the kinds data that is available to other organisations through the use of "Data Sharing Policies". For example one organisation may not allow another organisation to retrieve stock quantities, but may allow the retrieving organisation to get product pricing data. Data sharing policies may also be used to restrict the kinds of products that another organisation may retrieve.
The endpoint allows a maximum of 5000 records to be retrieved in one request. Where there are more than 5000 records to retrieve the calling software can use the "records_start_index" and "records_max_amount" parameters to step through and download the a range of records across multiple requests.
Before using this endpoint we recommend that you understand what the Ecommerce Standards Documents are and how you can read data from the standards into your own business logic. Note that there are trading token costs to retrieve the organisation data using this API. These costs may be paid by the organisation supplying the data, or the organisation retrieving the data depending on the data sharing policy that the supplying organisation has set up. See Trading Tokens and Pricing for information on costs.
HTTP Request
HTTP Method | GET | |
HTTP URL | https://api.squizz.com/rest/1/org/retrieve_esd/session_id |
|
Parameters | Data Type | Mandatory |
---|---|---|
session_id | STRING | Yes |
ID of the API session. Place the session ID within the URL | ||
supplier_org_id | STRING | Yes |
ID of the organisation issued by the platform to retrieve the data from. | ||
data_type_id | INTEGER | Yes |
ID of the type of data to retrieve. Set one of the numbers:
|
||
records_start_index | INTEGER | No |
The index to start retrieving the records from. For example if there are 8000 product records, setting the record_start_index to 2000 will get records numbered 2000 onwards. If the parameter is not set then the start index is set to 0. | ||
records_max_amount | INTEGER | No |
Sets the maximum amount of records that are returned. For example if set to 1000 then only up to 1000 records will be returned by the endpoint. If the parameter is not set or the value is higher than 5000 then the parameter will be set to 5000. For Product Combinations the maximum amount of records is limited to 500 records. For Maker Model Mappings the maximum amount of records is limited to 100,000 records. | ||
customer_account_code | STRING | Conditional |
Code of the supplying organisation's customer account. The account code is only required when the "data_type_id" is set to 37 to retrieve Customer Account Product Pricing, and if the supplying organisation has assigned multiple customer accounts to the organisation calling the endpoint. The customer account code will dictate how the products are priced. | ||
records_updated_after_date_time | LONG INTEGER | No |
Filter to only obtain records that have been updated after the specified date time. Date time is provided in milliseconds since the 01/01/1970 12AM UTC epoch. For example to obtain records update after 16/03/2023 14:21:16 AEDT then provide the value: 1678936875000 This parameter can be used to reduce the amount of data being retrieved, to only records that changed since the last time the endpoint was called for the given data type requested. Note that Updated Date Time for a record may get updated, even if no data was altered for a record when the supplying organisation performed a data import that includes the record. Ensure that a positive number is provided, otherwise the parameter will be ignored and no records wil lbe filtered by updated date time. |
HTTP Response
Response Data Type | JSON |
Parameters | Data Type |
---|---|
result | ENUM (SUCCESS or FAILURE) |
Either "SUCCESS" or "FAILURE". If successful then the data was successfully retrieved. | |
resultStatus | INTEGER |
|
|
version | DECIMAL |
Version of the Ecommerce Standards Document being returned from the server. | |
configs.api_version | DECIMAL |
Version of the SQUIZZ.com platform's API used to handle the request | |
configs.result_code | STRING |
Status code of trying to retrieve the organisation's data. The following codes could be returned:
|
|
configs.recordsStartIndex | INTEGER |
The start index of the record that records were returned from | |
configs.recordsMaxAmount | INTEGER |
The maximum amount of records that have been returned by the endpoint | |
configs.dataFields | CSV |
Contains a list of comma separated values containing the fields that are included in the record data being exported. If the field is displayed in this CSV list but the record does not contain the field in the dataRecords array, then it denotes that the record is using the default value of the field. | |
dataRecords | JSON ARRAY |
List of records based on the data type set in the request:
|
HTTP Response Example:
Links
- Platform API Endpoint: Retrieve Organisation Data : Retrieve Maker data
- Platform API Endpoint: Retrieve Organisation Data : Retrieve Product Stock Quantity Data
- Platform API Endpoint: Retrieve Organisation Data : Retrieve Product Data
- Platform API Endpoint: Retrieve Organisation Data : Retrieve Category Data
- Platform API Endpoint: Retrieve Organisation Data : Retrieve Maker Model Mapping Data
- Platform API Endpoint: Retrieve Organisation Data : Retrieve Maker Model Data
- Platform API Endpoint: Retrieve Organisation Data : Retrieve Attributes and Product Attribute Value Data
- Platform API Endpoint: Retrieve Organisation Data : Retrieve Product Pricing Data
- Platform API Endpoint: Retrieve Organisation Data : Retrieve Product Combination Data
- Platform API Endpoint: Retrieve Organisation Data
Web Service Endpoint: Procure And Send Purchase Order To Supplier
The endpoint is used by a customer organisation to push one or more purchase orders into the platform and have it procured by a designated supplier organisation. "Procure" means for the customer organisation to find and buy goods/services off the supplying organisation. This endpoint allows a customer organisation to automate purchasing off selected supplier organisations registered in the platform. Additionally it helps a supplier organisations to automate selling to existing customer organisations.
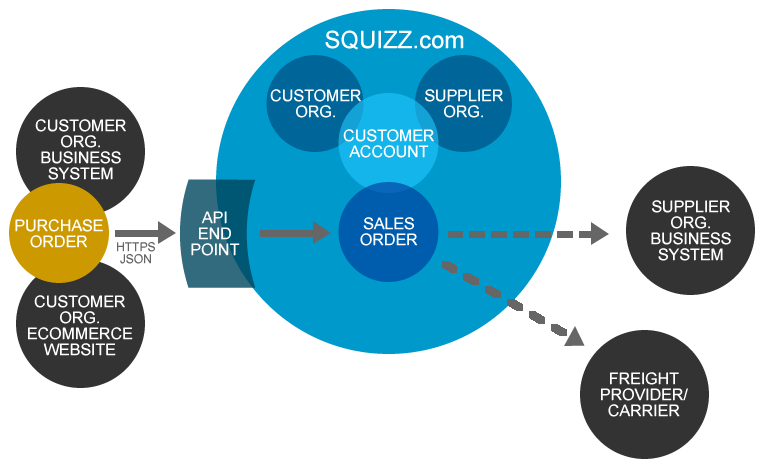
The endpoint will look through each purchase order given to it and try to find matching products that the chosen supplying organisation sells. Once all ordered products have been matched then the endpoint will price each product based on the quantities, relationship and customer account that the supplier organisation has assigned to the customer organisation. Only if all products in the purchase orders have been priced will the endpoint then create one or more sales orders for the supplier organisation and have the sales orders be marked as submitted for processing by the supplier organisation. The endpoint will then return each of the sales orders that were created, allowing the customer organisation to know the costs of the sales order and be able to update the purchase order based on the sales order totals.
Endpoint Requirements
To use this endpoint the following conditions need to be met:
- The customer organisation needs to be connected to the supplier organisation on the SQUIZZ.com platform.
- The supplier organisation's Trading Status must be set to Fully Trading or Selling Only.
- The customer organisation's Trading Status must be set to Fully Trading or Buying Only.
- The supplier organisation needs to have assigned at least one customer account to the customer organisation
- The assigned customer account needs to have an "On Account" payment type set, allowing the customer organisation to pay for the ordered products at a later date, based on agreed terms with the supplier organisation.
- The assigned customer account must be active and allow orders to be created against it (not on hold or outside terms and limits).
- Every product in the purchase orders must match to the supplying organisation's products based on either of the following:
- The customer's product code matches supplier's product code
- The customer's product is assigned to an alternate code that matches the supplier's product code, and the alternate codes have been previously imported into the platform by the customer organisation.
- The customer's product has previously been mapped to the supplier's product based on a previous order being submitted, either through this endpoint, or by a person using the Order Procurement feature within the platform.
- The supplier organisation is able to price all matched products in the purchase orders.
- The supplier organisation has enough trading tokens in the platform for the sales order transaction.
The purchase orders being imported must be placed into the Body of the HTTP request in the form of a conforming Ecommerce Standards Purchase Order Document JSON string, or otherwise an purchase order XML document in the xCBL version 3.0 standard. Before using this endpoint we recommend that you understand what the Ecommerce Standards Documents are and how you can convert purchase order data from your system into the standards document. It is highly recommended that any purchase order data uploaded using this endpoint be compressed using the GZIP compression algorithm. Note that there are trading token costs to the supplier organisation to have the sales orders created, of which the supplier organisation must have enough tokens to allow to do. For suppliers see the Trading Tokens and Pricing page for more details. The endpoint can be used without creating a session first, but then requires the customer organisation's api credentials to be passed across. It is recommended to create a session first before using this endpoint when multiple purchase orders need to be passed across, since it will reduce the amount of time required to authenticate each request.
HTTP Request
HTTP Method | POST | |
HTTP URL | https://api.squizz.com/rest/1/org/procure_purchase_order_from_supplier/session_id?supplier_org_id=[org_id]&customer_account_code=[customer_account_code] |
|
Headers | ||
---|---|---|
Content-Type | application/json | |
api-org-key | Conditional, only set this heading if create_single_request_session parameter is set to Y. Set it to the API key of the organisation sending the purchase order to authentic the request. | |
api-org-pw | Conditional, only set this heading if create_single_request_session parameter is set to Y. Set it to the API password of the organisation sending the purchase order to authentic the request. | |
Parameters | Data Type | Mandatory |
session_id | STRING | Yes |
ID of the API session. Place the session ID within the URLID of the API session. | ||
supplier_org_id | STRING | Yes |
ID of the organisation in the platform that is to supply the goods and services | ||
org_id | ID of the organisation that is sending the purchase order. This is only required if the create_single_request_session parameter is set to Y | Conditional |
customer_account_code | STRING | Conditional |
Code of the supplying organisation's customer account. The account code is only required if the supplying organisation has assigned multiple customer accounts to the customer organisation calling the endpoint, and the supplying organisation has not linked any supplier account codes or location codes set within the purchase order to their relevent customer account. See how supplying organisations link codes. | ||
create_single_request_session | ENUM(Y,N) | No |
If set to Y, then indicates that this endpoint is being called without any session being created first, and that api credentials have been passed in the HTTP request headers to authenticate the request. If the correct credentials are given then a session will be created for just the order import then once finished the session will be destroyed. This in effect allows systems to authenticate and send purchase orders in the same request, which may be required when systems have no way of retaining session data between HTTP requests. Note that this option will cause the request to take longer to process due to the additional authentication checks that need to be made. If this parameter is set to Y then the value of the session_id in the URL will be ignored. | ||
convert_from_document |
ENUM(XCBL3.0, CXML1.2, ANSIX12) |
No |
If set to XCBL3.0 then the contents of data placed into the body of the HTTP request will be treated as a purchase order in the XML file format conforming to the xCBL version 3.0 data format. Only set this parameter to XCBL3.0 if the system sending the purchase order has no ability to sending purchase orders in the Ecommerce standards, which is preferred data format. If set to CXML1.2 then the contents of data placed into the body of the HTTP request will be treated as a purchase order in the XML file format conforming to the cXML version 1.2 data format. Only set this parameter to CXML1.2 if the system sending the purchase order has no ability to sending purchase orders in the Ecommerce standards, which is preferred data format. Note that earlier and later versions of the cXML standard may be pushed, but limitated data types may be supported being converted. If this parameter is not set then the data in the body must conform to the Purchase Order Ecommerce Standards Document JSON format. Note that the endpoint will convert xCBL, cXML and ANSI x12 files into the Purchase Order Ecommerce Standards Document before processing occurs. See sections below about how this conversatoin takes place. |
||
response_http_error_code | INTEGER | No |
Set to a HTTP response status code that should be returned if any error occurs when calling the endpoint. The system calling this endpoint may require a non 200-OK response code to be returned in the response to identify a failed order procurement attempt. It is preferred for systems to parse the JSON response returned by this endpoint instead of using HTTP response status codes to determine if orders were successfully processed. This allows systems to more accurately report what error occurred. Where that is not possible then set the parameter in the URL. | ||
response_data_type | ENUM(ESD, CXML1.2) | No |
If this parameter is set to ESD or not set then the data returned in the endpoint's HTTP response will be JSON data conforming to the Sales Order Ecommerce Standards Document . The document will include the response status, response code, and the sales orders that were successfully created from the purchase orders given. This includes pricing data, any surcharges, and other relevant data. This is the preferred return type for this endpoint. If set to CXML1.2 then the data returned in the endpoint's HTTP response will have in its body XML formatted data conforming to the cXML Response 1.2 data standard, outlining if the endpoint successfully processed the purchase order(s) given. The response data will include the response code, and response status, but does not include any sales order information. The Payload ID will only be returned in the response if authentication succeeds first. Typically only set this parameter to CXML1.2 if the system calling this endpoint can only send and receive data in the cXML standard. |
||
set_unmatched_products_to_text_lines | ENUM(Y,N) | No |
If set to Y, then indicates that if a product line in the purchase order cannot be matched up to a supplying organisation's product (either due to no product mapping found, cannot be priced, or product is out of stock), then allow the product order line to be set as a text line instead. This then puts on the onus on the supplying organisation to determine how to handle a problematic product line, after the sales order has been created in the supplying organisation's own system. Subsequently this may lead to more human involvement from either/both trading organisations, with negotiation required to determine how to handle a product that cannot be found, priced, or stocked. This can add additional labour cost. For this behaviour to occur the supplying organisation must also allow this relaxed validation behaviour to occur through the Data Sharing Policy's "Allow Unmapped Sales Order Lines Set To Text Lines" permission set to Allow, that the purchasing organisation is assigned to. If both the permission and this parameter is set to Y, then it allows purchasing organisations to push through purchase orders with less order line validation. Note that the ideal approach is typically for the purchasing system that's pushing through the purchase order to handle any errors returned by this API endpoint when product lines cannot be matched, and using the error response to raise the order issue with purchasing staff, or modifying the order to remove problematic lines (the default behaviour). It is only advised to set this parameter to Y when the purchasing system does not have such a reporting/management capability, and the supplying organisation is willing to fix up orders on their end (at the cost of the purchasing system not immediately knowing the full price of the purchase order at the time of calling this API endpoint). |
||
Body | JSON or XML | Yes |
Body contains a Purchase Order Ecommerce Standards Document that has one or more purchase order records. The document is serialized into its JSON data string. If the convert_from_document parameter is set to Y then the body instead should be an XML purchase order conforming to the xCBL3.0 data format (note that is is not preferred due to the limitations of that data standard). |
To see more information about the purchase order fields that the endpoint supports you can find more information at Purchase Order Records Fields.
Example Raw HTTP Request
POST https://api.squizz.com/rest/1/org/procure_purchase_order_from_supplier/402934A8234E1B997D51F23974?supplier_org_id=342429EDABC3432498737 HTTP/1.1
Host: api.squizz.com
Content-Length: 3031
Content-Type: application/json
{
"resultStatus":"1",
"message":"The purchase order data has been successfully obtained.",
"configs":{},
"dataTransferMode": "COMPLETE",
"version": 1.1,
"totalDataRecords": 1,
"dataRecords":
[
{
"keyPurchaseOrderID":"111",
"purchaseOrderCode":"POEXAMPLE-345",
"purchaseOrderNumber":"345",
"keySupplierAccountID":"2",
"supplierAccountCode":"ACM-002",
"supplierAccountName":"Acme Supplies",
"currencyISOCode": "AUD",
"deliveryContact":"Jane Doe",
"deliveryOrgName":"Acme Industries",
"deliveryEmail":"js@someemailaddress.comm",
"deliveryPhone":"+6144433332222",
"deliveryFax":"+6144433332221",
"deliveryAddress1":"Unit 5",
"deliveryAddress2":"22 Bourkie Street",
"deliveryAddress3":"Melbourne",
"deliveryPostcode":"3000",
"deliveryRegionName":"Victoria",
"deliveryCountryName":"Australia",
"deliveryCountryCodeISO2":"AU",
"deliveryCountryCodeISO3":"AUS",
"billingContact":"John Citizen",
"billingOrgName":"Acme Industries International",
"billingEmail":"ms@someemailaddress.comm",
"billingPhone":"+61445242323423",
"billingFax":"+61445242323421",
"billingAddress1":"43",
"billingAddress2":"High Street",
"billingAddress3":"Melbourne",
"billingPostcode":"3000",
"billingRegionName":"Victoria",
"billingCountryName":"Australia",
"billingCountryCodeISO2":"AU",
"billingCountryCodeISO3":"AUS",
"instructions":"Leave goods at the back entrance",
"isDropship":"N",
"lines":
[
{
"lineType":"PRODUCT",
"quantity": 4,
"priceExTax": 5.00,
"priceIncTax": 5.50,
"priceTax": 0.50,
"priceTotalExTax": 20.00,
"priceTotalIncTax": 22.00,
"priceTotalTax": 2.00,
"unitName": "EACH",
"productCode": "TEA-TOWEL-GREEN",
"productName": "Green tea towel - 30 x 6 centimetres" ,
"salesOrderProductCode": "SUPPLIER-TOWEL-GRN"
},
{
"lineType":"PRODUCT",
"quantity": 2,
"priceExTax": 5.00,
"priceIncTax": 5.50,
"priceTax": 0.50,
"priceTotalExTax": 10.00,
"priceTotalIncTax": 11.00,
"priceTotalTax": 1.00,
"unitName": "EACH",
"productCode": "TEA-TOWEL-BLUE",
"productName": "Blue tea towel - 30 x 6 centimetres",
"salesOrderProductCode": "SUP-TEA-TOWEL-BLUE"
}
]
}
]
}
HTTP Response
Response Data Type | JSON or XML (XML if request parameter "response_data_type" is set to CXML1.2) |
Parameters | Data Type |
---|---|
result | ENUM (SUCCESS or FAILURE) |
Either "SUCCESS" or "FAILURE". If successful then the data was imported into the platform against the organsiation. | |
configs.api_version | DECIMAL |
Version of the SQUIZZ.com platform's API used to handle the request | |
configs.result_code | STRING |
Status code of trying to import the ESD document data. The following codes could be returned:
|
|
configs.orders_with_unmapped_lines | STRING |
Contains a comma delimited list of key value pairs (that each are colon delimited) that specifies the index of the purchase order record and the index of the line within the record that could not be matched to a supplier's product, or the customer organisation does not have permission to purchase. For example if this value was set to "1:2,1:3,4:0", Then it would specify that the 2nd purchase order record (index 1) within the dataRecords array of the purchase orders uploaded in the endpoint request has lines 3 and 4 that have unmapped products (line indexes 2 and 3), and for the 5th purchase order record (record index 4) the 1st order line has an unmapped product (line index 0). |
|
configs.orders_with_unpriced_lines | STRING |
Contains a comma delimited list of key value pairs (that each are colon delimited) that specifies the index of the purchase order record and the index of the line within the record that could not be priced for a supplier's product. This indicates that supplier products were found for the purchase order lines but that the supplier organisation had not set any pricing of the products, based on the customer account that that the customer organisation was assigned to. For example if this value was set to "2:1,2:3,5:0", Then it would specify that the 3rd purchase order record (index 2) within the dataRecords array of the purchase orders uploaded in the endpoint request has lines 2 and 4 that have unpriced products (line indexes 1 and 3), and for the 6th purchase order record (record index 5) the 1st order line has an un-priced product (line index 0). |
|
configs.orders_with_unstocked_lines | STRING |
Contains a comma delimited list of key value pairs (that each are colon delimited) that specifies the index of the purchase order record and the index of the line within the record that were out of stock for a supplier's product. This indicates that supplier products were found for the purchase order lines but that the supplier organisation had no stock available for the products listed this attribute. For example if this value was set to "2:1,2:3,5:0", Then it would specify that the 3rd purchase order record (index 2) within the dataRecords array of the purchase orders uploaded in the endpoint request has lines 2 and 4 that have un-stocked products (line indexes 1 and 3), and for the 6th purchase order record (record index 5) the 1st order line has an un-stocked product (line index 0). |
|
dataRecords | JSON ARRAY |
List of Sales Order Ecommerce Standards Records. Each record contains details of the corresponding supplier's sales order that was generated from each purchase order. These details include sales order number, order line pricing, surcharge prices (such as freight and shipping) |
HTTP Response Example:
Web Service Endpoint: Import Organisation Sales Order
The endpoint is used by an organisation to push one or more sales orders into the SQUIZZ.com platform and have it validated, optionally repriced, any sales order rules applied against it, and send across to connected freight and business systems.
This endpoint can be used by an organisation to have its sales orders that are raised across multiple selling systems, such as Ecommerce websites, 3rd party marketplaces, customer relationship management (CRM) systems, and any other business systems that create orders, have those orders flow through the SQUIZZ.com platform, perform the neccessary order validation, then have those sales orders be feed back into the back end system(s) that are already integrated into the SQUIZZ.com platform. This may reduce the amount of complexity by using existing business system integrations, saving time and money, whilst providing more automation. If a sales order is successfully imported, the endpoint will return a sales order containing the most up to date and accurate order data, including pricing data, product data, surcharge data, location data, and payment data.
Note that this endpoint should not be used by customer organisations to push through orders, since otherwise this would give permission for customers to set any pricing they wished for the sales orders, as well as other security issues. Instead customer organisations should use the Procure And Send Purchase Order To Supplier endpoint to push through their purchase orders, that can be correctly validated and priced based on their relationship to the supplying organisation.
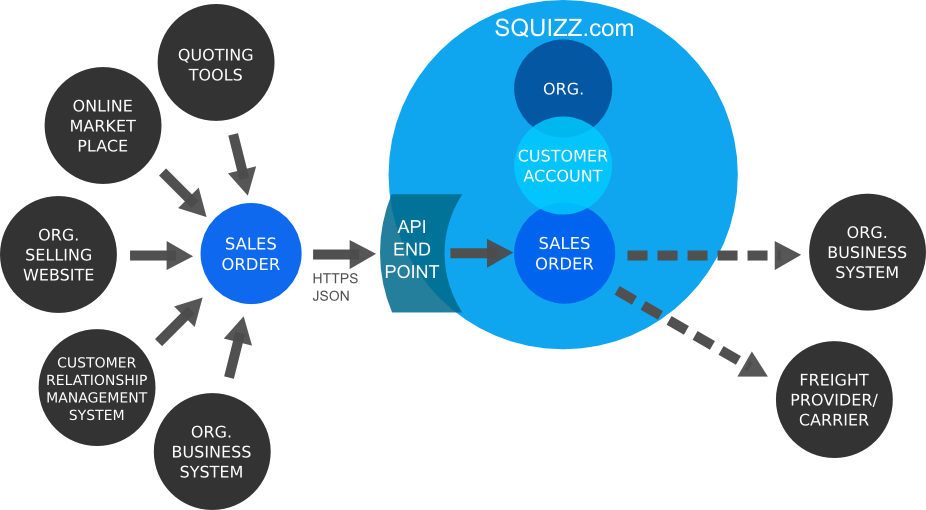
The endpoint will look through each sales order given to it and try to find matching products that the organisation sells, as well as matching up the surcharges, locations, payment types, and customer account. Once all order data have been matched then if required the endpoint will try to reprice each product based on the quantities, sell unit and customer account assigned to the order. If repricing is not required then the endpoint will check that all product lines and surcharges have had all pricing set. Only if all products and surcharges in the sales orders have been priced, and all other data matched will the endpoint then create one or more sales orders for the organisation and have the sales orders be marked as submitted for processing by the supplier organisation. Note that the sales order rules will also be run, allowing additional surcharges location data, and freight providers to be optionally applied to the order. If successfultThe endpoint will then return each of the sales orders that were created, allowing the requesting system to know the costs of the sales order and be able to update the sales order based on the sales order totals.
Endpoint Requirements
To use this endpoint the following conditions need to be met:
- The organisation needs to have previously imported the customer accounts into SQUIZZ.com that sales orders are being imported against
- The organisation needs have previously imported taxcodes, sell units, products, price-levels, price-level pricing/customer account pricing into SQUIZZ.com, that allows products to be validated and priced for the sales order product lines.
- If surcharges need to be applied against the order then the organisation needs to have previously imported surcharges into SQUIZZ.com. If the surcharges need to be repriced then sales order rules need to have been previously set up that specify when a surcharge should be applied against sales orders, and how the surcharges are priced.
- The organisation needs to have previously imported payment types into SQUIZZ.com, based on the payment types set within the sales order.
- The assigned customer account must be active and allow orders to be created against it
- Every product in the sales orders must match the organisation's products based on either of the following:
- The sales order product line's Product Code must match either a previously imported product's Product Code
- The sales order product line's Key Product ID must match either a previously imported product's Product Code
- If the imported sales order needs to be repriced then the pricing for the product must be previously imported. This includes price-level pricing that is assigned to the customer account that the order is associated to, or any customer account specific pricing for the order's customer account.
- If the imported sales order does not need to be repriced then the sales order product lines priceExTax, priceIncTax, PriceTax, totalPriceExTax, totalPriceIncTax and totalPriceTax fields must have values set.
- If the imported sales order does not need to be repriced then the sales order surcharges need to have price values set for the priceExTax, priceIncTax and priceTax values.
- The sales order's currency must match the organisation's currency configured against the Organisation Settings.
- The organisation must have enough trading tokens in the platform to cover the sales order transaction.
The sales orders being imported must be placed into the Body of the HTTP request in the form of a conforming Ecommerce Standards Sales Order Document JSON string. Before using this endpoint we recommend that you understand what the Ecommerce Standards Documents are and how you can send sales order data from your system into the standards document. It is highly recommended that any sales order data uploaded using this endpoint be compressed using the GZIP compression algorithm. Note that there are trading token costs to the organisation to have the sales orders imported, of which the organisation must have enough tokens to allow to do. See the Trading Tokens and Pricing page for more details.
HTTP Request
HTTP Method | POST | |
HTTP URL | https://api.squizz.com/rest/1/org/import_sales_order_esd/session_id?reprice_order=[reprice_order] | |
Headers | ||
---|---|---|
Content-Type | application/json | |
api-org-key | Conditional, only set this heading if create_single_request_session parameter is set to Y. Set it to the API key of the organisation sending the purchase order to authenticate the request. | |
api-org-pw | Conditional, only set this heading if create_single_request_session parameter is set to Y. Set it to the API password of the organisation sending the purchase order to authenticate the request. | |
Parameters | Data Type | Mandatory |
session_id | STRING | Conditional |
ID of the API session. Place the session ID within the URL. Must be set if the create_single_request_session parameter is not set to Y. | ||
reprice_order | ENUM(Y, N) | No |
If set to Y (Yes) then all product lines and surcharges in the sales order(s) will be repriced. If set to N (No) then the endpoint will check that all mandatory pricing fields in the sales order, product lines and surcharges have been provided. | ||
create_single_request_session | ENUM(Y,N) | No |
If set to Y, then indicates that this endpoint is being called without any session being created first, and that api credentials have been passed in the HTTP request headers to authenticate the request. If the correct credentials are given then a session will be created for just the order import then once finished the session will be destroyed. This in effect allows systems to authenticate and send sales orders in the same request, which may be required when systems have no way of retaining session data between HTTP requests. Note that this option will cause the request to take longer to process due to the additional authentication checks that need to be made. If this parameter is set to Y then the value of the session_id in the URL will be ignored. | ||
response_http_error_code | INTEGER | No |
Set to a HTTP response status code that should be returned if any error occurs when calling the endpoint. The system calling this endpoint may require a non 200-OK response code to be returned in the response to identify a failed sales order import attempt. It is preferred for systems to parse the JSON response returned by this endpoint instead of using HTTP response status codes to determine if orders were successfully processed. This allows systems to more accurately report what error occurred. Where that is not possible then set the parameter in the URL. | ||
Body | JSON | Yes |
Body contains a Sales Order Ecommerce Standards Document that has one or more sales order records. The document is serialized into its JSON data string. |
To see more information about the sales order fields that the endpoint supports you can find more information at Sales Order Records Fields.
Example Raw HTTP Request
POST https://api.squizz.com/rest/1/org/import_sales_order_esd/402934A8234E1B997D51F23974?reprice_order=N HTTP/1.1
Host: api.squizz.com
Content-Length: 3031
Content-Type: application/json
{
"resultStatus":"1",
"message":"The sales order data has been successfully obtained.",
"configs":{},
"dataTransferMode": "COMPLETE",
"version": 1.1,
"totalDataRecords": 1,
"dataRecords":
[
{
"keySalesOrderID":"111",
"salesOrderCode":"SOEXAMPLE-123",
"salesOrderNumber":"345",
"keyCustomerAccountID":"3",
"customerAccountCode":"ACM-002",
"customerAccountName":"Acme Supplies",
"currencyISOCode": "AUD",
"deliveryContact":"Jane Doe",
"deliveryOrgName":"Acme Industries",
"deliveryEmail":"js@someemailaddress.comm",
"deliveryPhone":"+6144433332222",
"deliveryFax":"+6144433332221",
"deliveryAddress1":"Unit 5",
"deliveryAddress2":"22 Bourkie Street",
"deliveryAddress3":"Melbourne",
"deliveryPostcode":"3000",
"deliveryRegionName":"Victoria",
"deliveryCountryName":"Australia",
"deliveryCountryCodeISO2":"AU",
"deliveryCountryCodeISO3":"AUS",
"billingContact":"John Citizen",
"billingOrgName":"Acme Industries International",
"billingEmail":"ms@someemailaddress.comm",
"billingPhone":"+61445242323423",
"billingFax":"+61445242323421",
"billingAddress1":"43",
"billingAddress2":"High Street",
"billingAddress3":"Melbourne",
"billingPostcode":"3000",
"billingRegionName":"Victoria",
"billingCountryName":"Australia",
"billingCountryCodeISO2":"AU",
"billingCountryCodeISO3":"AUS",
"instructions":"Leave goods at the back entrance",
"isDropship":"N",
"lines":
[
{
"lineType":"PRODUCT",
"quantity": 4,
"priceExTax": 5.00,
"priceIncTax": 5.50,
"priceTax": 0.50,
"priceTotalExTax": 20.00,
"priceTotalIncTax": 22.00,
"priceTotalTax": 2.00,
"unitName": "EACH",
"productCode": "TEA-TOWEL-GREEN",
"productName": "Green tea towel - 30 x 6 centimetres"
},
{
"lineType":"PRODUCT",
"quantity": 2,
"priceExTax": 5.00,
"priceIncTax": 5.50,
"priceTax": 0.50,
"priceTotalExTax": 10.00,
"priceTotalIncTax": 11.00,
"priceTotalTax": 1.00,
"unitName": "EACH",
"productCode": "TEA-TOWEL-BLUE",
"productName": "Blue tea towel - 30 x 6 centimetres"
}
]
}
]
}
HTTP Response
Response Data Type | JSON |
Parameters | Data Type |
---|---|
result | ENUM (SUCCESS or FAILURE) |
Either "SUCCESS" or "FAILURE". If successful then the sales order data was imported into the platform against the organsiation. | |
configs.api_version | DECIMAL |
Version of the SQUIZZ.com platform's API used to handle the request | |
configs.result_code | STRING |
Status code of trying to import the ESD document data. The following codes could be returned:
|
|
configs.orders_with_unmapped_lines | STRING |
Contains a comma delimited list of key value pairs (that each are colon delimited) that specifies the index of the sales order record and the index of the line within the record that could not be matched to a organisation's product. For example if this value was set to "1:2,1:3,4:0", Then it would specify that the 2nd sales order record (index 1) within the dataRecords array of the sales orders uploaded in the endpoint request has lines 3 and 4 that have unmatched products (line indexes 2 and 3), and for the 5th sales order record (record index 4) the 1st order line has an unmatched product (line index 0). |
|
configs.orders_with_unpriced_lines | STRING |
Contains a comma delimited list of key value pairs (that each are colon delimited) that specifies the index of the sales order record and the index of the line within the record that could not be priced for a organisation's product. This indicates that products were found for the sales order lines but that the organisation had not set any pricing of the products, based on the customer account that that the customer organisation was assigned to, or otherwise not all pricing fields were set if the order is not to be repriced. For example if this value was set to "2:1,2:3,5:0", Then it would specify that the 3rd sales order record (index 2) within the dataRecords array of the sales orders uploaded in the endpoint request has lines 2 and 4 that have unpriced products (line indexes 1 and 3), and for the 6th sales order record (record index 5) the 1st order line has an un-priced product (line index 0). |
|
configs.orders_with_unmatched_surcharges | STRING |
Contains a comma delimited list of key value pairs (that each are colon delimited) that specifies the index of the sales order record and the index of the surcharge record within the order record that could not be matched to a organisation's surcharge. For example if this value was set to "1:2,1:3,4:0", Then it would specify that the 2nd sales order record (index 1) within the dataRecords array of the sales orders uploaded in the endpoint request has surcharge records 3 and 4 that have unmatched surcharge (surcharge record indexes 2 and 3), and for the 5th sales order record (record index 4) the 1st order surcharge record has an unmatched surcharge (surcharge record index 0). |
|
configs.orders_with_unpriced_surcharges | STRING |
Contains a comma delimited list of key value pairs (that each are colon delimited) that specifies the index of the sales order record and the index of the surcharge within the record that could not be priced for a organisation's surcharge. This indicates that not all pricing fields were set against the surcharge if the order is not to be repriced. For example if this value was set to "2:1,2:3,5:0", Then it would specify that the 3rd sales order record (index 2) within the dataRecords array of the sales orders uploaded in the endpoint request has surcharge records 2 and 4 that have unpriced surcharges (surcharge indexes 1 and 3), and for the 6th sales order record (record index 5) the 1st order surcharge record has an un-priced surcharge (line index 0). |
|
configs.orders_with_unmatched_payments | STRING |
Contains a comma delimited list of key value pairs (that each are colon delimited) that specifies the index of the sales order record and the index of the payment record within the order record that could not be matched to a organisation's payment type. For example if this value was set to "1:2,1:3,4:0", Then it would specify that the 2nd sales order record (index 1) within the dataRecords array of the sales orders uploaded in the endpoint request has payment records 3 and 4 that have unmatched payment type (payment record indexes 2 and 3), and for the 5th sales order record (record index 4) the 1st order payment record has an unmatched payment (payment record index 0). |
|
dataRecords | JSON ARRAY |
List of Sales Order Ecommerce Standards Records. Each record contains details of the corresponding sales order that was generated from each imported sales order. These details include sales order number, order line pricing, surcharge prices (such as freight and shipping) |
HTTP Response Example:
Web Service Endpoint: Send Customer Invoice To Customer
The endpoint is used by a supplier organisation to push one or more invoices into the platform and have it be passed to a customer organisation, we it can then be imported back into the customer's business system. This allows organisations who supply goods and services to other organisations to automate the invoicing process, save time and money for both suppliers and customers. When a supplier organisation sends a "customer invoice" through this API endpoint, it will convert the customer invoice into a supplier invoice, and if the purchaser organisation requires it the invoice's lines can be matched up to the purchasers products, surcharges and taxcodes. This may then allow the invoice to be imported into the purchaser organisation's business system with its own codes.
HTTP Request
HTTP Method | POST | |
HTTP URL | https://api.squizz.com/rest/1/org/send_customer_invoice_to_customer/session_id?customer_org_id=[org_id]&supplier_account_code=[supplier_account_code] |
|
Headers | ||
---|---|---|
Content-Type | application/json | |
Parameters | Data Type | Mandatory |
session_id | STRING | Yes |
ID of the API session. Place the session ID within the URL | ||
customer_org_id | STRING | Yes |
ID of the organisation in the platform that is to recieve the invoice. | ||
supplier_account_code | STRING | No |
Code of the customer organisation's supplier account. The account code is only required if the customer organisation has assigned multiple supplier accounts (a.k.a. creditors or vendors) to the supplier organisation calling the endpoint. | ||
Body | JSON | Yes |
Body contains a Customer Invoice Ecommerce Standards Document that has one or more customer invoice records. The document is serialized into its JSON data string. |
To see more information about the customer invoice fields that the endpoint supports you can find more information at Customer Invoice Records Fields.
Example Raw HTTP Request
POST https://api.squizz.com/rest/1/org/send_customer_invoice_to_customer/402934A8234E1B997D51F23974?supplier_org_id=342429EDABC3432498737 HTTP/1.1
Host: api.squizz.com
Content-Length: 3031
Content-Type: application/json
{
"resultStatus":"1",
"message":"The customer invoice data has been successfully obtained.",
"configs":{},
"dataTransferMode": "COMPLETE",
"version": 1.1,
"totalDataRecords": 1,
"dataRecords":
[
{
"keyPurchaseOrderID":"111",
"purchaseOrderCode":"POEXAMPLE-345",
"purchaseOrderNumber":"345",
"keySupplierAccountID":"2",
"supplierAccountCode":"ACM-002",
"supplierAccountName":"Acme Supplies",
"currencyISOCode": "AUD",
"deliveryContact":"Jane Doe",
"deliveryOrgName":"Acme Industries",
"deliveryEmail":"js@someemailaddress.comm",
"deliveryPhone":"+6144433332222",
"deliveryFax":"+6144433332221",
"deliveryAddress1":"Unit 5",
"deliveryAddress2":"22 Bourkie Street",
"deliveryAddress3":"Melbourne",
"deliveryPostcode":"3000",
"deliveryRegionName":"Victoria",
"deliveryCountryName":"Australia",
"deliveryCountryCodeISO2":"AU",
"deliveryCountryCodeISO3":"AUS",
"billingContact":"John Citizen",
"billingOrgName":"Acme Industries International",
"billingEmail":"ms@someemailaddress.comm",
"billingPhone":"+61445242323423",
"billingFax":"+61445242323421",
"billingAddress1":"43",
"billingAddress2":"High Street",
"billingAddress3":"Melbourne",
"billingPostcode":"3000",
"billingRegionName":"Victoria",
"billingCountryName":"Australia",
"billingCountryCodeISO2":"AU",
"billingCountryCodeISO3":"AUS",
"instructions":"Leave goods at the back entrance",
"isDropship":"N",
"lines":
[
{
"lineType":"PRODUCT",
"quantity": 4,
"priceExTax": 5.00,
"priceIncTax": 5.50,
"priceTax": 0.50,
"priceTotalExTax": 20.00,
"priceTotalIncTax": 22.00,
"priceTotalTax": 2.00,
"unitName": "EACH",
"productCode": "TEA-TOWEL-GREEN",
"productName": "Green tea towel - 30 x 6 centimetres" ,
"salesOrderProductCode": "SUPPLIER-TOWEL-GRN"
},
{
"lineType":"PRODUCT",
"quantity": 2,
"priceExTax": 5.00,
"priceIncTax": 5.50,
"priceTax": 0.50,
"priceTotalExTax": 10.00,
"priceTotalIncTax": 11.00,
"priceTotalTax": 1.00,
"unitName": "EACH",
"productCode": "TEA-TOWEL-BLUE",
"productName": "Blue tea towel - 30 x 6 centimetres",
"salesOrderProductCode": "SUP-TEA-TOWEL-BLUE"
}
]
}
]
}
HTTP Response
Response Data Type | JSON |
Parameters | Data Type |
---|---|
result | ENUM (SUCCESS or FAILURE) |
Either "SUCCESS" or "FAILURE". If successful then the data was imported into the platform against the organsiation. | |
configs.api_version | DECIMAL |
Version of the SQUIZZ.com platform's API used to handle the request | |
configs.result_code | STRING |
Status code of trying to import the ESD document data. The following codes could be returned:
|
|
configs.invoices_with_unmapped_lines | STRING |
Contains a comma delimited list of key value pairs (that each are colon delimited) that specifies the index of the customer invoice record and the index of the line within the record that could not be matched to a customer's product. For example if this value was set to "1:2,1:3,4:0", Then it would specify that the 2nd customer invoice record (index 1) within the dataRecords array of the invoices uploaded in the endpoint request has lines 3 and 4 that have unmapped products (line indexes 2 and 3), and for the 5th customer invoice record (record index 4) the 1st order line has an unmapped product (line index 0). |
|
configs.invoices_with_unmapped_line_taxcodes | STRING |
Contains a comma delimited list of key value pairs (that each are colon delimited) that specifies the index of the customer invoice record and the index of the line within the record that contains a taxcode that could not be matched to to one of the customer's taxcodes. For example if this value was set to "1:2,1:3,4:0", Then it would specify that the 2nd customer invoice record (index 1) within the dataRecords array of the invoices uploaded in the endpoint request has lines 3 and 4 that have unmapped taxcodes (line indexes 2 and 3), and for the 5th customer invoice record (record index 4) the 1st order line has an unmapped taxcode (line index 0). |
|
configs.invoices_with_unmapped_surcharges | STRING |
Contains a comma delimited list of key value pairs (that each are colon delimited) that specifies the index of the customer invoice record and the index of the surcharge within the record that could not be matched to a customer's surcharge. For example if this value was set to "1:2,1:3,4:0", Then it would specify that the 2nd customer invoice record (index 1) within the dataRecords array of the invoices uploaded in the endpoint request has surcharges 3 and 4 that have unmapped surcharges (surcharge line indexes 2 and 3), and for the 5th customer invoice record (record index 4) the 1st order surcharge has an unmapped surcharge (surcharge line index 0). |
|
configs.invoices_with_unmapped_surcharge_taxcodes | STRING |
Contains a comma delimited list of key value pairs (that each are colon delimited) that specifies the index of the customer invoice record and the index of the surcharge that contains a taxcode that could not be matched to a customer's taxcode. For example if this value was set to "1:2,1:3,4:0", Then it would specify that the 2nd customer invoice record (index 1) within the dataRecords array of the invoices uploaded in the endpoint request has surcharges 3 and 4 that have unmapped taxcodes for surcharges (surcharge line indexes 2 and 3), and for the 5th customer invoice record (record index 4) the 1st order surcharge has an unmapped surcharge taxcode (surcharge line index 0). |
|
dataRecords | JSON ARRAY |
List of Supplier Invoice Ecommerce Standards Records. Each record contains details of the corresponding customer's supplier invoice that was generated from each customer invoice. These details include supplier invoice number, invoice line pricing, surcharge prices (such as freight and shipping) |
HTTP Response Example:
Web Service Endpoint: Send Delivery Notice To Customer
The endpoint is used by a supplier organisation to push one or more delivery notices into the platform and have it be raised against a customer account, and alerted by the purchasing person who raised the original order, as well as be passed to a customer organisation. The delivery notice can then be optionally imported back into the customer's business system. This allows organisations who supply goods to alert their customers of the status and progress of the delivery(s). This also customer organisations to automate recieving notices of future and expected deliveries, save time and money for both suppliers and customers. When a supplier organisation sends a "delivery notice" through this API endpoint, it will be first assigned against the customer account its associated, as well the sales order if one already exists in squizz. If a matching sales order is found then if the order was raised for an individual person then they will receive a notification advising of the delivery notice. If the order was raised by a customer organisation, or the API request specifies the customer organisation who is to receive the notice, then the notice will be sent to the customer organisation, where it can then be optionally exported back into their own system.
HTTP Request
HTTP Method | POST | |
HTTP URL | https://api.squizz.com/rest/1/org/send_delivery_notice_to_customer/session_id?customer_org_id=[org_id]&supplier_account_code=[supplier_account_code]&use_delivery_notice_export=[Y,N] |
|
Headers | ||
---|---|---|
Content-Type | application/json | |
Parameters | Data Type | Mandatory |
session_id | STRING | Yes |
ID of the API session. Place the session ID within the URL | ||
customer_org_id | STRING | No |
ID of the organisation in the platform that is to recieve the delivery notice. | ||
supplier_account_code | STRING | No |
Code of the customer organisation's supplier account. The account code is only required if the customer organisation has assigned multiple supplier accounts (a.k.a. creditors or vendors) to the supplier organisation calling the endpoint. This is used to determine which supplier account in the customer's system should the delivery notice be raised against. | ||
use_delivery_notice_export | ENUM (Y or N) | No |
If set to Y, then after the delivery notice is assigned to the customer account in SQUIZZ, it will then be attempted to be exported to another external system using the default data adaptor of the supplying organisation and its Export Customer Delivery Notice data export. This is typically only used if the customer isn't able to integrate their system into squizz, and relies upon the supplier organisation to push the data to a nominated system, or a 3rd party system such as a Marketplace. | ||
Body | JSON | Yes |
Body contains a General Ecommerce Standards Document that indicates if the data was succesfully processed. The document is serialized into its JSON data string. |
To see more information about the customer invoice fields that the endpoint supports you can find more information at Delivery Notice Records Fields.
Example Raw HTTP Request
POST https://api.squizz.com/rest/1/org/send_delivery_notice_to_customer/402934A8234E1B997D51F23974?supplier_org_id=342429EDABC3432498737 HTTP/1.1
Host: api.squizz.com
Content-Length: 3031
Content-Type: application/json
{
"resultStatus":"1",
"message":"The delivery notice data has been successfully obtained.",
"configs":{},
"dataTransferMode": "COMPLETE",
"version": 1.1,
"totalDataRecords": 1,
"dataRecords":
[
{
"keyPurchaseOrderID":"111",
"purchaseOrderCode":"POEXAMPLE-345",
"purchaseOrderNumber":"345",
"keySupplierAccountID":"2",
"supplierAccountCode":"ACM-002",
"supplierAccountName":"Acme Supplies",
"currencyISOCode": "AUD",
"deliveryContact":"Jane Doe",
"deliveryOrgName":"Acme Industries",
"deliveryEmail":"js@someemailaddress.comm",
"deliveryPhone":"+6144433332222",
"deliveryFax":"+6144433332221",
"deliveryAddress1":"Unit 5",
"deliveryAddress2":"22 Bourkie Street",
"deliveryAddress3":"Melbourne",
"deliveryPostcode":"3000",
"deliveryRegionName":"Victoria",
"deliveryCountryName":"Australia",
"deliveryCountryCodeISO2":"AU",
"deliveryCountryCodeISO3":"AUS",
"billingContact":"John Citizen",
"billingOrgName":"Acme Industries International",
"billingEmail":"ms@someemailaddress.comm",
"billingPhone":"+61445242323423",
"billingFax":"+61445242323421",
"billingAddress1":"43",
"billingAddress2":"High Street",
"billingAddress3":"Melbourne",
"billingPostcode":"3000",
"billingRegionName":"Victoria",
"billingCountryName":"Australia",
"billingCountryCodeISO2":"AU",
"billingCountryCodeISO3":"AUS",
"instructions":"Leave goods at the back entrance",
"isDropship":"N"
}
]
}
HTTP Response
Response Data Type | JSON |
Parameters | Data Type |
---|---|
result | ENUM (SUCCESS or FAILURE) |
Either "SUCCESS" or "FAILURE". If successful then the data was imported into the platform against the organsiation. | |
configs.api_version | DECIMAL |
Version of the SQUIZZ.com platform's API used to handle the request | |
configs.result_code | STRING |
Status code of trying to import the ESD document data. The following codes could be returned:
|
HTTP Response Example:
Web Service Endpoint: Create Organisation Notification
The endpoint allows an organisation notification to be created in the platform and sent to the relevant people assigned to the organisation's notification category. This endpoint allows external systems to raise notifications in the platform containing a tailored message. This endpoint could be used to alert organisation people of events happening outside of the platform that warrents their attention, such as events happening within a website, business system, or other software. These notifications may also be sent as push notifications to mobile devices that have the Squizz.com app installed. Note that there are trading token costs to create organisation notifications, of which the organisation must have enough tokens to allow the notification to be raised. See the Trading Tokens and Pricing document for costs.
HTTP Request
HTTP Method | POST | |
HTTP URL | https://api.squizz.com/rest/1/org/create_notification/session_id |
|
Parameters | Data Type | Mandatory |
---|---|---|
session_id | STRING | Yes |
ID of the API session. Place the session ID within the URL | ||
notify_category | STRING | Yes |
Organisation category to place the notification within. Set one of the values:
|
||
message | STRING | Yes |
Message to place into the notification. All characters are treated a literals, however you may embed up to 5 links or labels into the message using the placeholder text formats:
|
||
link1_url | STRING | No |
URL of the link to place in the notification message where the placeholder {1} has been set | ||
link1_label | STRING | No |
label to place in the notification message where {1} is set. This label may be set as a link if link1_url has been set. | ||
link2_url | STRING | No |
URL of the link to place in the notification message where the placeholder {2} has been set | ||
link2_label | STRING | No |
label to place in the notification message where {2} is set. This label may be set as a link if link2_url has been set. | ||
link3_url | STRING | No |
URL of the link to place in the notification message where the placeholder {3} has been set | ||
link3_label | STRING | No |
label to place in the notification message where {3} is set. This label may be set as a link if link3_url has been set. | ||
link4_url | STRING | No |
URL of the link to place in the notification message where the placeholder {4} has been set | ||
link4_label | STRING | No |
label to place in the notification message where {4} is set. This label may be set as a link if link4_url has been set. | ||
link5_url | STRING | No |
URL of the link to place in the notification message where the placeholder {5} has been set | ||
link5_label | STRING | No |
label to place in the notification message where {5} is set. This label may be set as a link if link5_url has been set. |
HTTP Response
Response Data Type | JSON |
Parameters | Data Type |
---|---|
result | ENUM (SUCCESS or FAILURE) |
Either "SUCCESS" or "FAILURE". If successful then the notification was created. | |
result_code | STRING |
Status code of trying to create the notification. See the Appendix I for the list of possible result codes. | |
api_version | STRING |
Version of the API being used to handle the endpoint request. |
HTTP Response Example:
Web Service Endpoint: Validate Organisation Security Certificate
The endpoint validates an TLS/SSL x509 security certificate that was created in the organisation's profile. The source IP address of the API HTTP request is validated against the IP address or related domain (via DNS lookup) set in the certificate. If the checks pass then the security certificate becomes active.This endpoint is used to make sure that security certificates are being issued against legitimate public IP addresses or domains that the organisation is in control of.
HTTP Request
HTTP Method | POST | |
HTTP URL | https://api.squizz.com/rest/1/org/validate_cert/session_id |
|
Parameters | Data Type | Mandatory |
---|---|---|
session_id | STRING | Yes |
ID of the API session. Place the session ID within the URL | ||
org_security_certificate_id | STRING | Yes |
ID of the security certificate created in the organisation's profile |
HTTP Response
Response Data Type | JSON |
Parameters | Data Type |
---|---|
result | ENUM (SUCCESS or FAILURE) |
Either "SUCCESS" or "FAILURE". If successful then the certificate was validated. | |
result_code | STRING |
Status code of trying to validate the certificate. See the Appendix I for the list of possible result codes. | |
api_version | STRING |
Version of the API being used to handle the endpoint request. |
HTTP Response Example:
Web Service Endpoint: Search Organisation Customer Account Records
The SQUIZZ.com platform's API has an endpoint that allows an organisation to search for records within another connected organisation's business system, based on records associated to an assigned customer account. This may also be known as "Customer Account Enquiry" This endpoint allows an organisation to search for invoice, sales order, back order, transactions. credit and payment records, retrieved in realtime from a supplier organisation's connected business system. The records returned from endpoint are formatted as JSON data conforming to the "Ecommerce Standards Document" standards, with each document containing an array of zero or more records. The list of records found and returned is controlled by the supplying organisation's business system performing the search. This endpoint will return a list of records containing the overall details of each record. To retrieve the full details and any associated lines for each record call the "Organisation Retrieve Customer Account Record" endpoint.
Notes
- A session must first be created in the API before calling the endpoint.
- The organisation calling the endpoint must be connected to the "supplying" organisation that is facilitating the record searching.
- The organisation calling the endpoint must be assigned to a customer account by the supplying organisation.
- A maximum of 100 search requests can be made against a supplying organisation within the last 15 minutes. This ensures the supplying organisation is not flooded with too many requests.
- It's recommended that the supplying organisation's returning the records may be sorting the records by the "most recent date created" by default. However some organisation systems may not be able to sort this way, with records appearing with no specific sorting.
- The supplying organisation being called to perform the record search must have enough trading tokens to allow the searches to occur, as outlined in the Trading Tokens and Pricing document.
- The supplying organisation being called to the perform the record search must have set up an integration with their own business system(s) to SQUIZZ.com, containing the customer account record data. This system must support receiving requests made by the SQUIZZ.com platform, as outlined in the Outgoing Request: Get Customer Account Enquiry Records endpoint. The SQUIZZ.com Connector software can be used by the supplying organisation to facilitate searching in their own business system. if the system does not provide its own web service or support the Ecommece Standards Documents standard.
HTTP Method | GET | |
HTTP URL | https://api.squizz.com/rest/1/org/search_customer_account_records_esd/session_id |
|
Headers | Data Type | Mandatory |
---|---|---|
Content-Type | STRING | Yes |
application/json | ||
Parameters | Data Type | Mandatory |
session_id | STRING | Yes |
ID of the API session. Place the session ID within the URL | ||
supplier_org_id | STRING | Yes |
ID of the organisation on the SQUIZZ.com platform to obtain records from. | ||
customer_account_code | STRING | Yes |
Code of the supplying organisation's customer account that records are associated to. The organisation calling this API endpoint must be assigned to this customer account by the supplying organisation. | ||
record_type | STRING | Yes |
Type of records to obtain. Set to one of the following:
|
||
begin_date_time | LONG | No |
Datetime to obtain records from. Datetime is set as milliseconds since the 01-01-1970 UTC epoch. If not set then it will default to 90 days before the current date and time. Ensure this date time occurs before the end_date_time parameter's value. | ||
end_date_time | LONG | No |
Datetime to obtain records to. Datetime is set as milliseconds since the 01-01-1970 UTC epoch. If not set then it will default to to the current date and time. | ||
pageNumber | INTEGER | No |
The page number to display records on. By default it is set to page 1. | ||
records_max_amount | INTEGER | No |
Number of records to display per page. By default it is set to 100 | ||
outstanding_records | ENUM(true,false) | No |
If true then only obtain records that are outstanding or unpaid. Ignore if not given or empty. Typically only set to true when retrieving invoice records and wish to obtain invoices that are outstanding, not fully paid, or overdue. | ||
key_record_ids | STRING | No |
Comma delimited list of records to find based on each record's keyRecordID. Ignore if not given or empty. | ||
search_string | STRING | No |
Text used to search and match record data on. The field to search on is based on the search type given. If no value has been given against the search_type parameter then the records may be searched against the default field that the supplying business system wishes to use. Typically this would be the record's number or code, such as invoiceNumber, salesOrderNumber, paymentNumber etc... But this may vary between systems. | ||
search_type | STRING | No |
The field within the records to search and match the search string on. Ignored if not given or empty. Note that not all record fields are supported by the supplying organisation systems to search on. This may vary per business system. The common search fields are: |
HTTP Response
Response Data Type | JSON |
Response Body | Returns serialized JSON of a ESDocumentCustomerAccountEnquiry document containing a list of customer account records for the given record type. |
Parameters | Data Type |
---|---|
resultStatus | INTEGER |
If 1 then denotes a successful response has been returned. 0 or more records may have been found. If not 1 then indicates an error has occurred when trying to perform the search. | |
configs.result_code | ENUM (SUCCESS or FAILURE) |
Either "SUCCESS" or "FAILURE". If successful then the data was imported into the platform against the organsiation. | |
configs.api_version | DECIMAL |
Version of the SQUIZZ.com platform's API used to handle the request | |
configs.result_code | STRING |
Status code of trying to search for the customer account record data. The following codes could be returned:
|
|
configs.search_wait_period | STRING |
Amount of minutes that the organisation calling this endpoint needs to wait before being able to perform a search again. This is only relevant if the result is a failure. | |
invoiceRecords | JSON ARRAY |
List of Customer Account Enquiry Invoice Records Each record contains limited overall details about each invoice record found. It will be set to null unless the record_type request parameter is set to INVOICE. | |
transactionRecords | JSON ARRAY |
List of Customer Account Enquiry Transaction Records Each record contains limited overall details about each transaction record found. It will be set to null unless the record_type request parameter is set to TRANSACTION. | |
orderSaleRecords | JSON ARRAY |
List of Customer Account Enquiry Sales Order Records Each record contains limited overall details about each sales order record found. It will be set to null unless the record_type request parameter is set to ORDER_SALE. | |
backOrderRecords | JSON ARRAY |
List of Customer Account Enquiry Back Order Records Each record contains limited overall details about each back order record found. It will be set to null unless the record_type request parameter is set to BACKORDER. | |
creditRecords | JSON ARRAY |
List of Customer Account Enquiry Credit Records Each record contains limited overall details about each credit record found. It will be set to null unless the record_type request parameter is set to CREDIT. | |
paymentRecords | JSON ARRAY |
List of Customer Account Enquiry Payment Records Each record contains limited overall details about each payment record found.It will be set to null unless the record_type request parameter is set to PAYMENT. |
Native Coding Library Examples:
HTTP Response Example:
"resultStatus": 1,
"version":1.3,
"message": "",
"configs":{
"api_version":"1.0.0.0",
"result_status": "SUCCESS",
"result_code":"SERVER_SUCCESS",
"dataFields":"[csv_list_of_data_fields]"
},
"invoiceRecords":[list_containing_found_invoice_records],
"orderSaleRecords":[list_containing_found_sales_order_records],
"backOrderRecords":[list_containing_found_back_order_records],
"creditRecords":[list_containing_found_credit_records],
"paymentRecords":[list_containing_found_payment_records],
}
Links
- Platform API Endpoint: Search Organisation Customer Account Records : Search Payment Customer Account Records
- Platform API Endpoint: Search Organisation Customer Account Records
- Platform API Endpoint: Search Organisation Customer Account Records : Search Invoice Customer Account Records
- Platform API Endpoint: Search Organisation Customer Account Records : Search Transaction Customer Account Records
- Platform API Endpoint: Search Organisation Customer Account Records : Search Sales Order Customer Account Records
- Platform API Endpoint: Search Organisation Customer Account Records : Search Back Order Customer Account Records
- Platform API Endpoint: Search Organisation Customer Account Records : Search Credit Customer Account Records
Web Service Endpoint: Retrieve Organisation Customer Account Record
The SQUIZZ.com platform's API's "Retrieve Organisation Customer Account Record" endpoint allows an organisation to retrieve the details and lines for a record from another connected organisation's business sytem, based on a record associated to an assigned customer account. This endpoint allows an organisation to securely get the details for a invoice, sales order, back order, transactions. credit or payment record, retrieved in realtime from a supplier organisation's connected business system. The record returned from endpoint is formatted as JSON data conforming to the "Ecommerce Standards Document" standards, with the document containing an array of zero or one record. The list of records found and returned is controlled by the supplying organisation's business system performing the search. This endpoint will return a list of records containing the overall details of each record. To retrieve the full details and any associated lines for each record call the "Organisation Retrieve Customer Account Record" endpoint.
Notes
- A session must first be created in the API before calling the endpoint.
- The organisation calling the endpoint must be connected to the "supplying" organisation that is facilitating the record searching.
- The organisation calling the endpoint must be assigned to a customer account by the supplying organisation.
- A maximum of 100 search requests can be made against a supplying organisation within the last 15 minutes. This ensures the supplying organisation is not flooded with too many requests.
- The supplying organisation being called to perform the record search must have enough trading tokens to allow the searches to occur, as outlined in the Trading Tokens and Pricing document.
- The supplying organisation being called to the perform the record search must have set up an integration with their own business system(s) to SQUIZZ.com, containing the customer account record data. This system must support receiving requests made by the SQUIZZ.com platform, as outlined in the Outgoing Request: Get Customer Account Enquiry Record endpoint. The SQUIZZ.com Connector software can be used by the supplying organisation to facilitate searching in their own business system. if the system does not provide its own web service or support the Ecommece Standards Documents standards.
HTTP Method | GET | |
HTTP URL | https://api.squizz.com/rest/1/org/retrieve_customer_account_record_esd/session_id |
|
Headers | Data Type | Mandatory |
---|---|---|
Content-Type | STRING | Yes |
application/json | ||
Parameters | Data Type | Mandatory |
session_id | STRING | Yes |
ID of the API session. Place the session ID within the URL | ||
supplier_org_id | STRING | Yes |
ID of the organisation on the SQUIZZ.com platform to obtain records from. | ||
customer_account_code | STRING | Yes |
Code of the supplying organisation's customer account that records are associated to. The organisation calling this API endpoint must be assigned to this customer account by the supplying organisation. | ||
record_type | STRING | Yes |
ID of type of record to obtain. Set to one of the following:
|
||
key_record_id | STRING | Yes |
The unique key identifier of the record to retrieve. This identifier can be found by calling the Search Organisation Customer Account Records API endpoint. For the different record type being retrieved set this value, based on the value set in one if the record's fields found from the Search Organisation Customer Account Records API endpoint:
|
HTTP Response
Response Data Type | JSON |
Response Body | ESDocumentCustomerAccountEnquiry Returns serialized JSON of a ESDocumentCustomerAccountEnquiry document containing 0 or 1 records within the list of customer account records for the given record type. |
Parameters | Data Type |
---|---|
resultStatus | INTEGER |
If 1 then denotes a successful response has been returned. 0 or 1 record may have been found. If not 1 then indicates an error has occurred when trying to retrieve the record. | |
configs.result_status | ENUM (SUCCESS or FAILURE) |
Either "SUCCESS" or "FAILURE". If successful then the data was retrieved from the supplying organsiation's business system. | |
configs.api_version | DECIMAL |
Version of the SQUIZZ.com platform's API used to handle the request | |
configs.result_code | STRING |
Status code of trying to search for the customer account record data. The following codes could be returned:
|
|
configs.search_wait_period | STRING |
Amount of minutes that the organisation calling this endpoint needs to wait before being able to perform a retrieval again. This is only relevant if the result is a failure. | |
invoiceRecords | JSON ARRAY |
List of Customer Account Enquiry Invoice Records containing 0 or 1 records with the found invoice record. This array will only be not null if the record_type is set to INVOICE, | |
orderSaleRecords | JSON ARRAY |
List of Customer Account Enquiry Sales Order Records containing 0 or 1 records with the found sales order record. This array will only be not null if the record_type is set to ORDER_SALE | |
backOrderRecords | JSON ARRAY |
List of Customer Account Enquiry Back Order Records containing 0 or 1 records with the found back order record. This array will only be not null if the record_type is set to BACKORDER | |
creditRecords | JSON ARRAY |
List of Customer Account Enquiry Credit Records containing 0 or 1 records with the found credit record. This array will only be not null if the record_type is set to CREDIT | |
paymentRecords | JSON ARRAY |
List of Customer Account Enquiry Payment Records containing 0 or 1 records with the found payment record. This array will only be not null if the record_type is set to PAYMENT |
HTTP Response Example:
"resultStatus": 1,
"version":1.3,
"message": "",
"configs":{
"api_version":"1.0.0.0",
"result_status": "SUCCESS",
"result_code":"SERVER_SUCCESS",
"dataFields":"[csv_list_of_data_fields]"
},
"invoiceRecords":[list_containing_found_invoice_record],
"orderSaleRecords":[list_containing_found_sales_order_record],
"backOrderRecords":[list_containing_found_back_order_record],
"creditRecords":[list_containing_found_credit_record],
"paymentRecords":[list_containing_found_payment_record],
}
Links
- Platform API Endpoint: Retrieve Organisation Customer Account Record
- Platform API Endpoint: Retrieve Organisation Customer Account Record : Retrieve Invoice Customer Account Record
- Platform API Endpoint: Retrieve Organisation Customer Account Record : Retrieve Sales Order Customer Account Record
- Platform API Endpoint: Retrieve Organisation Customer Account Record : Retrieve Back Order Customer Account Record
- Platform API Endpoint: Retrieve Organisation Customer Account Record : Retrieve Credit Customer Account Record
- Platform API Endpoint: Retrieve Organisation Customer Account Record : Retrieve Payment Customer Account Record
Outgoing Request: Get Customer Account Enquiry Records
The Squizz.com platform can send out a request to an organisation's connected business system to request a list of records associated to a customer account in the organisation's business system. This allows data such as invoices, sales orders, back orders, credits, payments, and transactions to be all queried in real time, and filtered over a certain date range. The platform will only make requests to obtain this customer account data if the person is connected to the organisation and has permission to request the data based on their relationship to a customer account, or the search request is initiated from the Search Customer Account Records.organisation API endpoint.This request can be used to help a person or organisation to find one or more records that they are looking for within a supplying organisation's system.
HTTP Request
HTTP Method | GET | ||
HTTP URL | https://[ipaddress_domain] |
||
Request Header | Data Type | Mandatory | Description |
---|---|---|---|
Accept-Encoding | STRING | Yes | Set to gzip-deflate. Responses from the webservice/connector should compress data to reduce the download times. |
Authorization | STRING | Yes | Contains a base64 encoded string containing the username and password configured in the organisation's profile admin section, in the Connector Settings dialog.This is used to authenticate that the incoming request from Squizz.com is allowed to be processed by the webservice, using Basic Authentication. |
Squizz-Org-ID | STRING | Yes | ID of the organisation issued by squizz.comThis may be optionally used to further authenticate the incoming request from Squizz.com. |
Parameters | Data Type | Mandatory | Description |
ipaddress_domain | STRING | Yes | The public IP address or domain used to call the organisation's designated webservice/Connector. Set within the URL. This can be configured in the Admin area of an organisation's profile, within the Connector settings dialog. |
port | STRING | Yes | The port number to make the request to against the organisation's designated webservice/Connector. Set within the URL. This can be configured in the Admin area of an organisation's profile, within the Connector settings dialog. |
keyCustomerAccountID | STRING | Yes | Unique ID of the organisation's customer account |
recordType | STRING | Yes | ID of type of records to obtain. Set to one of the following:
|
beginDate | LONG | No | Datetime to obtain records from. Datetime is set as milliseconds since the 01-01-1970 UTC epoch |
endDate | LONG | No | Datetime to obtain records to. Datetime is set as milliseconds since the 01-01-1970 UTC epoch |
pageNumber | INTEGER | Yes | The page number to display records on. |
numberOfRecords | INTEGER | Yes | Number of records to display per page. |
outstandingRecords | ENUM | No | If true then only obtain records that are outstanding or unpaid. Ignore if not given or empty |
keyRecordIDs | STRING | No | Comma delimited list of records to find based on each record's keyRecordID. Ignore if not given or empty. |
searchString | STRING | No | text to match record data on. The field to search on is based on the search type given. Ignore if the searchType has not been given or is empty. |
searchType | STRING | No | The field within the records to search and match the search string on. Ignore if not given or empty. |
orderByField | STRING | No | The field to order the records by. If not given or empty then sort by the record's date field. |
orderByDirection | ENUM (DESC or ASC) | No | The direction to order the records by. Set to either DESC-descending or ASC-ascending. By default order by descending |
HTTP Response
Response Data Type | JSON | |
Response Body | ESDocumentCustomerAccountEnquiry | |
---|---|---|
Returns serialized JSON of a ESDocumentCustomerAccountEnquiry document containing a list of customer account records for the given record type. |
Outgoing Request: Get Customer Account Enquiry Record
The Squizz.com platform can send out a request to an organisation's connected business system to request the details of a single record associated to a customer account in the organisation's business system. This allows data such as an invoice, sales order, back order, credit or payment record to be queried in real time. The platform will only make requests to obtain this customer account record data if the person is connected to the organisation and has permission to request the data based on their relationship to a customer account.
HTTP Request
HTTP Method | GET | ||
HTTP URL | https://[ipaddress_domain] |
||
Request Header | Data Type | Mandatory | Description |
---|---|---|---|
Accept-Encoding | STRING | Yes | Set to gzip-deflate. Responses from the webservice/connector should compress data to reduce the download times. |
Authorization | STRING | Yes | Contains a base64 encoded string containing the username and password configured in the organisation's profile admin section, in the Connector Settings dialog.This is used to authenticate that the incoming request from Squizz.com is allowed to be processed by the webservice, using Basic Authentication. |
Squizz-Org-ID | STRING | Yes | ID of the organisation issued by squizz.comThis may be optionally used to further authenticate the incoming request from Squizz.com. |
Parameters | Data Type | Mandatory | Description |
ipaddress_domain | STRING | Yes | The public IP address or domain used to call the organisation's designated webservice/Connector. Set within the URL. This can be configured in the Admin area of an organisation's profile, within the Connector settings dialog. |
port | STRING | Yes | The port number to make the request to against the organisation's designated webservice/Connector. Set within the URL. This can be configured in the Admin area of an organisation's profile, within the Connector settings dialog. |
keyCustomerAccountID | STRING | Yes | Unique ID of the organisation's customer account |
recordType | STRING | Yes | ID of type of record to obtain. Set to one of the following:
|
keyRecordID | STRING | Yes | Unique ID of the customer account record to obtain. |
HTTP Response
Response Data Type | JSON | |
Response Body | ESDocumentCustomerAccountEnquiry | |
---|---|---|
Returns serialized JSON of a ESDocumentCustomerAccountEnquiry document containing a single record in the records list for the given record type. |
Outgoing Request: Get Supplier Account Enquiry Records
The Squizz.com platform can send out a request to an organisation's connected business system to request a list of records associated to a supplier account in the organisation's business system. This allows data such as purchase orders to be all queried in real time, and filtered over a certain date range. The platform will only make requests to obtain this supplier account data if the person is connected to the organisation and has permission to request the data based on their relationship to a supplier account. This request can be used to help a person find one or more records that they are looking for, which can be used to then convert purchase orders into sales orders associated to a supplier organisation.
HTTP Request
HTTP Method | GET | ||
HTTP URL | https://[ipaddress_domain] |
||
Request Header | Data Type | Mandatory | Description |
---|---|---|---|
Accept-Encoding | STRING | Yes | Set to gzip-deflate. Responses from the webservice/connector should compress data to reduce the download times. |
Authorization | STRING | Yes | Contains a base64 encoded string containing the username and password configured in the organisation's profile admin section, in the Connector Settings dialog.This is used to authenticate that the incoming request from Squizz.com is allowed to be processed by the webservice, using Basic Authentication. |
Squizz-Org-ID | STRING | Yes | ID of the organisation issued by squizz.comThis may be optionally used to further authenticate the incoming request from Squizz.com. |
Parameters | Data Type | Mandatory | Description |
ipaddress_domain | STRING | Yes | The public IP address or domain used to call the organisation's designated webservice/Connector. Set within the URL. This can be configured in the Admin area of an organisation's profile, within the Connector settings dialog. |
port | STRING | Yes | The port number to make the request to against the organisation's designated webservice/Connector. Set within the URL. This can be configured in the Admin area of an organisation's profile, within the Connector settings dialog. |
keySupplierAccountID | STRING | Yes | Unique ID of the organisation's supplier account |
recordType | STRING | Yes | ID of type of records to obtain. Set to one of the following:
|
beginDate | LONG | No | Datetime to obtain records from. Datetime is set as milliseconds since the 01-01-1970 UTC epoch |
endDate | LONG | No | Datetime to obtain records to. Datetime is set as milliseconds since the 01-01-1970 UTC epoch |
pageNumber | INTEGER | Yes | The page number to display records on. |
numberOfRecords | INTEGER | Yes | Number of records to display per page. |
outstandingRecords | ENUM | No | If true then only obtain records that are outstanding or unpaid. Ignore if not given or empty |
keyRecordIDs | STRING | No | Comma delimited list of records to find based on each record's keyRecordID. Ignore if not given or empty. |
searchString | STRING | No | text to match record data on. The field to search on is based on the search type given. Ignore if the searchType has not been given or is empty. |
searchType | STRING | No | The field within the records to search and match the search string on. Ignore if not given or empty. |
orderByField | STRING | No | The field to order the records by. If not given or empty then sort by the record's date field. |
orderByDirection | ENUM (DESC or ASC) | No | The direction to order the records by. Set to either DESC-descending or ASC-ascending. By default order by descending |
HTTP Response
Response Data Type | JSON | |
Response Body | ESDocumentSupplierAccountEnquiry | |
---|---|---|
Returns serialized JSON of a ESDocumentSupplierAccountEnquiry document containing a list of customer account records for the given record type. |
Outgoing Request: Get Supplier Account Enquiry Record
The Squizz.com platform can send out a request to an organisation's connected business system to request the details of a single record associated to a supplier account in the organisation's business system. This allows data such as a purchase order record to be queried in real time. The platform will only make requests to obtain this supplier account record data if the person is connected to the organisation and has permission to request the data based on their relationship to a supplier account.
HTTP Request
HTTP Method | GET | ||
HTTP URL | https://[ipaddress_domain] |
||
Request Header | Data Type | Mandatory | Description |
---|---|---|---|
Accept-Encoding | STRING | Yes | Set to gzip-deflate. Responses from the webservice/connector should compress data to reduce the download times. |
Authorization | STRING | Yes | Contains a base64 encoded string containing the username and password configured in the organisation's profile admin section, in the Connector Settings dialog.This is used to authenticate that the incoming request from Squizz.com is allowed to be processed by the webservice, using Basic Authentication. |
Squizz-Org-ID | STRING | Yes | ID of the organisation issued by squizz.comThis may be optionally used to further authenticate the incoming request from Squizz.com. |
Parameters | Data Type | Mandatory | Description |
ipaddress_domain | STRING | Yes | The public IP address or domain used to call the organisation's designated webservice/Connector. Set within the URL. This can be configured in the Admin area of an organisation's profile, within the Connector settings dialog. |
port | STRING | Yes | The port number to make the request to against the organisation's designated webservice/Connector. Set within the URL. This can be configured in the Admin area of an organisation's profile, within the Connector settings dialog. |
keySupplierAccountID | STRING | Yes | Unique ID of the organisation's supplier account |
recordType | STRING | Yes | ID of type of record to obtain. Set to one of the following:
|
keyRecordID | STRING | Yes | Unique ID of the supplier account record to obtain. |
HTTP Response
Response Data Type | JSON | |
Response Body | ESDocumentSupplierAccountEnquiry | |
---|---|---|
Returns serialized JSON of a ESDocumentSupplierAccountEnquiry document containing a single record in the records list for the given record type. |
Outgoing Request: Post Purchase Order
The Squizz.com platform can send out a request to an organisation's connected business system to post a purchase order. This allows purchasers who have imported a purchase order into the platform to submit the purchase order back to the organisation's system without needing to do manual data entry. The platform will only make requests to post a purchase order if the person is connected to the organisation and has permission to post purchase orders.
HTTP Request
HTTP Method | POST | ||
HTTP URL | https://[ipaddress_domain] |
||
Request Header | Data Type | Mandatory | Description |
---|---|---|---|
Accept-Encoding | STRING | Yes | Set to gzip-deflate. Responses from the webservice/connector should compress data to reduce the download times. |
Authorization | STRING | Yes | Contains a base64 encoded string containing the username and password configured in the organisation's profile admin section, in the Connector Settings dialog.This is used to authenticate that the incoming request from Squizz.com is allowed to be processed by the webservice, using Basic Authentication. |
Squizz-Org-ID | STRING | Yes | ID of the organisation issued by squizz.comThis may be optionally used to further authenticate the incoming request from Squizz.com. |
Parameters | Data Type | Mandatory | Description |
ipaddress_domain | STRING | Yes | The public IP address or domain used to call the organisation's designated webservice/Connector. Set within the URL. This can be configured in the Admin area of an organisation's profile, within the Connector settings dialog. |
port | STRING | Yes | The port number to make the request to against the organisation's designated webservice/Connector. Set within the URL. This can be configured in the Admin area of an organisation's profile, within the Connector settings dialog. |
Request Body | JSON | Yes | Serialized form of the ESDocumentOrderPurchase document containing one ESDRecordOrderPurchase record in the JSON. |
HTTP Response
Response Data Type | JSON | |
Response Body | ESDocument | |
---|---|---|
Returns serialized JSON of a ESDocument with the resultStatus property specifying if the order successfully imported or not. |
Outgoing Request: Post Sales Order
The Squizz.com platform can send out a request to an organisation's connected business system to post a sales order. This allows sales orders that have been created in the platform by customers to submit the sales order back to the organisation's system without needing to do manual data entry. The platform will only make requests to post a sales order if the person is connected to the organisation and has permission to post sales orders, or otherwise a sales order has been created through the platform's API by an organisation who has permission to raise sales orders from purchase orders that they have submitted.
HTTP Request
HTTP Method | POST | ||
HTTP URL | https://[ipaddress_domain] |
||
Request Header | Data Type | Mandatory | Description |
---|---|---|---|
Accept-Encoding | STRING | Yes | Set to gzip-deflate. Responses from the webservice/connector should compress data to reduce the download times. |
Authorization | STRING | Yes | Contains a base64 encoded string containing the username and password configured in the organisation's profile admin section, in the Connector Settings dialog.This is used to authenticate that the incoming request from Squizz.com is allowed to be processed by the webservice, using Basic Authentication. |
Squizz-Org-ID | STRING | Yes | ID of the organisation issued by squizz.comThis may be optionally used to further authenticate the incoming request from Squizz.com. |
Parameters | Data Type | Mandatory | Description |
ipaddress_domain | STRING | Yes | The public IP address or domain used to call the organisation's designated webservice/Connector. Set within the URL. This can be configured in the Admin area of an organisation's profile, within the Connector settings dialog. |
port | STRING | Yes | The port number to make the request to against the organisation's designated webservice/Connector. Set within the URL. This can be configured in the Admin area of an organisation's profile, within the Connector settings dialog. |
Request Body | JSON | Yes | Serialized form of the ESDocumentOrderSale document containing one ESDRecordOrderSale record in the JSON. |
HTTP Response
Response Data Type | JSON | |
Response Body | ESDocument | |
---|---|---|
Returns serialized JSON of a ESDocument with the resultStatus property specifying if the order successfully imported or not. |
Outgoing Request: Post Customer Account Invoice Payment
The Squizz.com platform can send out a request to an organisation's connected business system to post a payment made against an invoice for a given customer account.This allows customers to pay for invoices through the platform, then have the payment automatically posted back against the invoice record in the organisation's business system.
HTTP Request
HTTP Method | POST | ||
HTTP URL | https://[ipaddress_domain] |
||
Request Header | Data Type | Mandatory | Description |
---|---|---|---|
Accept-Encoding | STRING | Yes | Set to gzip-deflate. Responses from the webservice/connector should compress data to reduce the download times. |
Authorization | STRING | Yes | Contains a base64 encoded string containing the username and password configured in the organisation's profile admin section, in the Connector Settings dialog.This is used to authenticate that the incoming request from Squizz.com is allowed to be processed by the webservice, using Basic Authentication. |
Squizz-Org-ID | STRING | Yes | ID of the organisation issued by squizz.comThis may be optionally used to further authenticate the incoming request from Squizz.com. |
Parameters | Data Type | Mandatory | Description |
ipaddress_domain | STRING | Yes | The public IP address or domain used to call the organisation's designated webservice/Connector. Set within the URL. This can be configured in the Admin area of an organisation's profile, within the Connector settings dialog. |
port | STRING | Yes | The port number to make the request to against the organisation's designated webservice/Connector. Set within the URL. This can be configured in the Admin area of an organisation's profile, within the Connector settings dialog. |
Request Body | JSON | Yes | Serialized form of the ESDocumentCustomerAccountPayment document containing one ESDRecordCustomerAccountPayment record in the JSON. |
HTTP Response
Response Data Type | JSON | |
Response Body | ESDocument | |
---|---|---|
Returns serialized JSON of a ESDocument with the resultStatus property specifying if the payment successfully imported or not. |
Outgoing Request: Get Connector Status
The Squizz.com platform can send out a request to an organisation's connected software to check its status and to test that the credentials to access it are correct. This endpoint can be called from within the organisation's profile, under the admin section, from within the Connector Settings dialog by clicking on the Test Connection button.
HTTP Request
HTTP Method | GET | ||
HTTP URL | https://[ipaddress_domain] |
||
Request Header | Data Type | Mandatory | Description |
---|---|---|---|
Accept-Encoding | STRING | Yes | Set to gzip-deflate. Responses from the webservice/connector should compress data to reduce the download times. |
Authorization | STRING | Yes | Contains a base64 encoded string containing the username and password configured in the organisation's profile admin section, in the Connector Settings dialog.This is used to authenticate that the incoming request from Squizz.com is allowed to be processed by the webservice, using Basic Authentication. |
Squizz-Org-ID | STRING | Yes | ID of the organisation issued by squizz.comThis may be optionally used to further authenticate the incoming request from Squizz.com. |
Parameters | Data Type | Mandatory | Description |
ipaddress_domain | STRING | Yes | The public IP address or domain used to call the organisation's designated webservice/Connector. Set within the URL. This can be configured in the Admin area of an organisation's profile, within the Connector settings dialog. |
port | STRING | Yes | The port number to make the request to against the organisation's designated webservice/Connector. Set within the URL. This can be configured in the Admin area of an organisation's profile, within the Connector settings dialog. |
HTTP Response
Response Data Type | JSON | |
Response Body | ESDocument | |
---|---|---|
Returns serialized JSON of a ESDocument with the resultStatus and message properties specifying if the status of the webservice is running OK. |